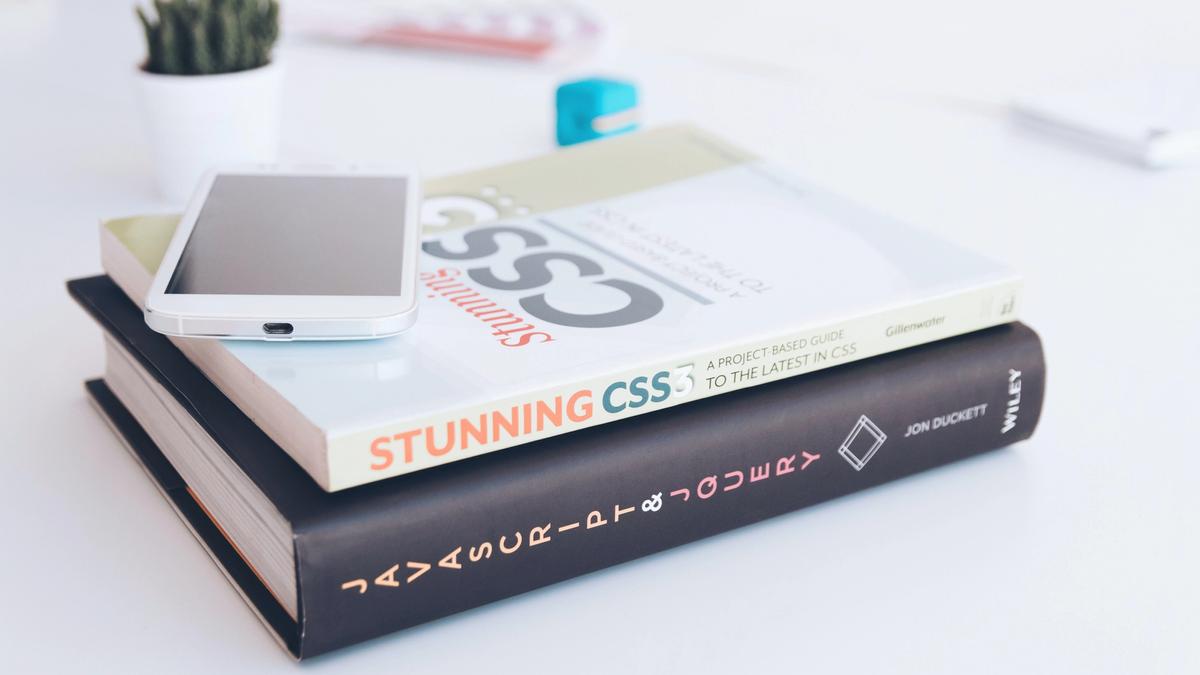
CSS pseudo elements and nth child
As the MDN web docs state, "a CSS pseudo-element is a keyword added to a selector that lets you style a specific part of the selected element(s)."
There are quite a few, but by far my most used are ::before and ::after.
The scenario I'm going to be talking about today came up when I was tasked to style up a page to mimic a design with both content and styling changes, but ONLY access to the CSS.
I was given absolutely no control over the HTML for... various reasons.
So the question was... is it even possible?
Well, on inspecting the HTML, I found many divs that I could utilise (just enough if all were used with ::before and ::after elements!) so that was possible. The only thing that couldn't be done is create an anchor element, so once that expectation was adjusted, I set to work creating all the pseudo elements I would need.
Before and after elements
To create a pseudo element in CSS, you'll need to add ::before or ::after to the normal selector. You'll also need to ensure there is "content", even if it is empty, otherwise the element won't display.
.classSelector::before {
content: '';
}
Another great tip is positioning. If you position the main element as relative, and the pseudo element as absolute, then the pseudo element will be positioned relative to the main element. So positioning the pseudo element as in the example below, the top left of both the main element and the attached pseudo element will align exactly.
.classSelector {
position: relative;
}
.classSelector::before {
content: '';
position: absolute;
top: 0;
left: 0;
}
Simply give it some content or a width and height and you'll have an element you can see and style.
Nth child selector
Another crucial part of this challenge was utilising CSS selectors (also known as pseudo classes). As I didn't have access to the HTML, I couldn't add classes, so I had to target certain classless and ID-less using selectors.
My most used ones are: first-child, last-child, and nth-child(x).
<div>First child</div>
<div>Second child</div>
<div>Third child</div>
<div>Last child</div>
So for the set of divs above, I would target them using the CSS below:
/* Selects any <div> that is the first element
among its siblings */
div:first-child {
font-weight: bold;
}
/* Selects the second <div> element */
div:nth-child(2) {
color: #000;
}
/* Selects every third <div> element */
div:nth-child(3n) {
color: #fff;
}
/* Selects any <div> that is the last element
among its siblings */
div:last-child {
text-decoration: underline;
}
Read more about pseudo classes here.
Using a combination of these techniques, I managed to recreate the client's design even without touching the HTML. It took some time, and obviously wasn't the ideal way, but given the project constraints, I feel was a very successful outcome.